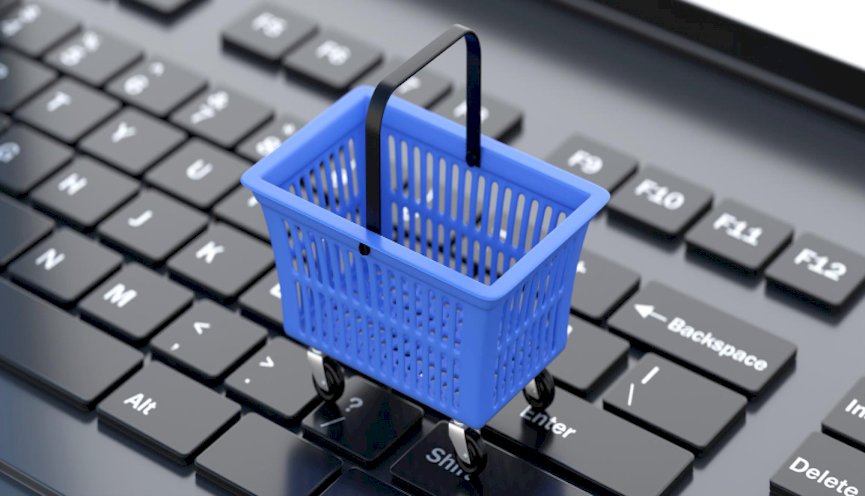
Recently we develped and deployed multi-vendor amazon clone built on react.js and node.js. Most difficult part was different tax rules of different countries and somewhat address Validations of various countries.
To build an Amazon clone application using Node.js and React.js, you will need to do the following:
-
Set up your development environment:
- Install Node.js and npm (Node Package Manager).
- Install a text editor (such as Visual Studio Code).
- Install create-react-app, a tool that allows you to quickly set up a React application with no configuration.
-
Create a new React application using create-react-app:
- Open a terminal and navigate to the directory where you want to create your application.
- Run the following command: npx create-react-app my-app. This will create a new directory called "my-app" with the basic structure of a React application.
-
Set up the back-end of your application:
- In the terminal, navigate to the root directory of your application (e.g. "my-app").
- Run the following command: npm init. This will create a "package.json" file, which will contain metadata about your project and a list of its dependencies.
- Install the following packages:
- express: a popular web framework for Node.js.
- mongoose: a library for working with MongoDB (a popular NoSQL database).
- body-parser: a library for parsing HTTP requests.
- Create a new file called "server.js" in the root directory of your application. This file will contain the code for your back-end server.
-
Set up the database:
- Install MongoDB on your computer or use a cloud service such as MongoDB Atlas.
- Connect to the database from your back-end server (e.g. in "server.js").
- Define the schema for your product data (e.g. name, price, etc.) and create a model using mongoose.
-
Create the API endpoints for your application:
- In "server.js", define the routes for your API using express.
- For each route, define the corresponding controller function that will handle the request and return the appropriate response.
- Examples of routes you may want to include are:
- GET /products: returns a list of all products in the database.
- POST /products: adds a new product to the database.
- GET /products/:id: returns a specific product by its ID.
- PUT /products/:id: updates a specific product by its ID.
- DELETE /products/:id: deletes a specific product by its ID.
-
Set up the front-end of your application:
- In the "src" directory of your React application, create a new directory called "components". This is where you will store the various React components for your application.
- Create a component for displaying a list of products (e.g. "ProductList.js").
- Create a component for displaying a single product (e.g. "Product.js").
- Create a component for adding a new product (e.g. "AddProduct.js").
- Use the fetch function to retrieve data from your API and display it in the appropriate components.
-
Add additional features and functionality as desired (e.g. user authentication, search functionality, etc.).
I hope this helps! Let me know if you have any questions or need further guidance.